Methods for the ListNode
class will consist of those for creating, accessing, and modifying nodes.
-
The constructor for the ListNode
class is responsible for creating a node and initializing the two instance variables of a new node.
public ListNode(Object initValue, ListNode initNext) {
// post: constructs a new element with object initValue,
// followed by next element
value = initValue;
next = initNext;
}
-
Here is an example of code to create the first node of a linked list.
ListNode first;
first = new ListNode(new Integer(23), null);
After execution of the two statements, first
refers to the header node of a small linked list that contains just one node with the Integer 23
.
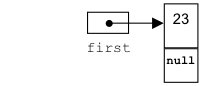
-
Getting and setting the data and link of the node are accomplished with getter and setter methods as follows.
public Object getValue(){
// post: returns value associated with this element
return value;
}
public ListNode getNext(){
// post: returns reference to next value in list
return next;
}
public void setValue(Object theNewValue) {
value = theNewValue;
}
public void setNext(ListNode theNewNext) {
// post: sets reference to new next value
next = theNewNext;
}
- The following segment of code using
ListNode
will illustrate the syntax of accessing the data members of a ListNode
.
ListNode list;
list = new ListNode(new Integer(13), null);
System.out.println("The node contains: " +
(Integer)list.getValue());
list.setValue(new Integer(17));
System.out.println("The node contains: " +
(Integer)list.getValue());
Run Output:
The node contains: 13
The node contains: 17